Pull request size plugin
This plugin adds the size information of pull requests to the pull request search page. It also adds the number of commits ahead and behind the destination branch.
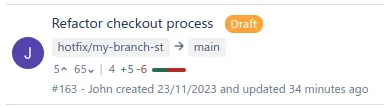
It’s enabled by default, but you can customize it further by:
- Categorizing pull requests by size thresholds
- Enforcing a maximum size threshold using a merge check
- Excluding files when calculating the size
Thresholds
You can define thresholds
for pull request changes to easily group and classify
them into different sizes and understand them at a glance. It also allows you to filter using the sizes you’ve defined.
function pullRequestSize(options: PullRequestSizeOptions): PluginDef<PullRequestSizeOptions> (+1 overload)
pullRequestSize({
PullRequestSizeOptions.thresholds?: Record<string, SizeCriteria> | undefined
thresholds: {
type Small: {
maxFiles: number;
maxTotalLines: number;
}
Small: {maxFiles?: number | undefined
maxFiles: 3, maxTotalLines?: number | undefined
maxTotalLines: 25},
type Medium: {
maxTotalLines: number;
}
Medium: {maxTotalLines?: number | undefined
maxTotalLines: 300},
type Large: {
maxLinesAdded: number;
maxLinesRemoved: number;
}
Large: {maxLinesAdded?: number | undefined
maxLinesAdded: 500, maxLinesRemoved?: number | undefined
maxLinesRemoved: 300},
type Huge: {}
Huge: {}, // <--- Catch all threshold
},
})
The names are arbitrary, and you can have as many thresholds as necessary. You
can use lines (maxTotalLines
,maxLinesAdded
,maxLinesRemoved
)
and files (maxFiles
) to define the threshold criteria.
Upper limits should usually be defined as a catch-all threshold. You can achieve this by using an empty criterion, as shown above.
Flowie automatically creates labels and associates them based on the thresholds.
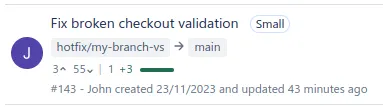
Enforce a maximum size
Once you have defined your thresholds,
you can optionally set a maximum size using the maxSize
option.
function pullRequestSize(options: PullRequestSizeOptions): PluginDef<PullRequestSizeOptions> (+1 overload)
pullRequestSize({
PullRequestSizeOptions.thresholds?: Record<string, SizeCriteria> | undefined
thresholds: {
type Small: {
maxTotalLines: number;
}
Small: {maxTotalLines?: number | undefined
maxTotalLines: 100},
type Medium: {
maxTotalLines: number;
}
Medium: {maxTotalLines?: number | undefined
maxTotalLines: 300},
type Large: {}
Large: {},
},
PullRequestSizeOptions.maxSize?: string | undefined
maxSize: "Medium",
})
In this example, pull requests classified above Medium
will fail the merge checks, thereby preventing them from being merged.
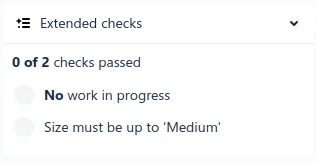
Excluding files
Some files or directories are generated and are usually not relevant during the code review. It’s possible to exclude these files when calculating the pull request size.
function pullRequestSize(options: PullRequestSizeOptions): PluginDef<PullRequestSizeOptions> (+1 overload)
pullRequestSize({
PullRequestSizeOptions.exclude?: string[] | undefined
exclude: ["package-lock.json"],
})
Complete example
import {const configure: (config: Config | (() => Config)) => void
configure} from "flowie.app"
import {const pullRequestSize: OptionsPluginNoDefault<PullRequestSizeOptions, false>
pullRequestSize, const merge: OptionsPlugin<MergePluginOptions, false>
merge} from "flowie.app/plugins"
function configure(config: Config | (() => Config)): void
configure({
Config.plugins?: PluginDef<unknown>[] | undefined
plugins: [
// Use merge plugin to enforce merge checks
function merge(): PluginDef<MergePluginOptions> (+2 overloads)
merge(),
function pullRequestSize(options: PullRequestSizeOptions): PluginDef<PullRequestSizeOptions> (+1 overload)
pullRequestSize({
PullRequestSizeOptions.thresholds?: Record<string, SizeCriteria> | undefined
thresholds: {
type Small: {
maxTotalLines: number;
}
Small: {maxTotalLines?: number | undefined
maxTotalLines: 100},
type Medium: {
maxTotalLines: number;
}
Medium: {maxTotalLines?: number | undefined
maxTotalLines: 300},
type Large: {}
Large: {},
},
PullRequestSizeOptions.maxSize?: string | undefined
maxSize: "Medium",
PullRequestSizeOptions.exclude?: string[] | undefined
exclude: ["package-lock.json"],
}),
],
})