Labels
Pull request labels allow you to categorize and filter pull requests. They are shown on the right side in the labels panel.
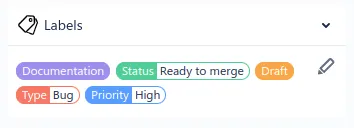
You can add or remove them by clicking on the edit (pencil) button.
You can define your own labels or use the default ones provided by Flowie. Plugins can also add their own labels.
Quick remove mode NEW
To enter quick remove mode, double-click on the labels panel and you should see the option to remove each label. Once you removed the labels you want, double-click again on the panel or click away from the panel to make the changes effective.
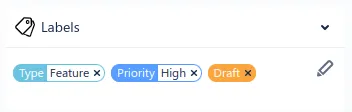
Defining labels
The labels are defined in the labels schema under the configuration property named labels
.
import {const configure: (config: Config | (() => Config)) => void
configure} from "flowie.app"
function configure(config: Config | (() => Config)): void
configure({
Config.labels?: {
schema?: Array<Label>;
} | undefined
labels: {
schema?: Label[] | undefined
schema: [{BasicLabel.name: string
name: "My label"}],
},
})
A label can be a BasicLabel
or LabelSet
.
BasicLabel
A BasicLabel
is the traditional
label that is used to tag.
{
BasicLabel.name: string
name: "Documentation",
LabelValueOptions.description?: string | undefined
description: "Documentation changes",
}
Results in:

LabelSet
LabelSet
is a label that has a name and can assume only a single
value from a set of values. It allows you to model custom workflows via status, for
example. It is also more convenient because you don’t to remove the old label when
you are changing the value. For instance, if you have a issue type label bug and
want to change it do feature, just replace assigning the feature value will remove
the bug.
{
BasicLabel.name: string
name: "Type",
LabelSet.values: (string | [string, LabelValueOptions & {
valueColor?: Color;
}])[]
values: ["Bug", "Feature"],
}
Results in:


Colors
You can also define the color of the label. The color is optional and if not provided, Flowie will use the default color. The color of the text is automatically determined based on the background color.
{
BasicLabel.name: string
name: "Type",
LabelValueOptions.color?: Color | undefined
color: "green",
LabelSet.values: (string | [string, LabelValueOptions & {
valueColor?: Color;
}])[]
values: ["Bug", "Feature"],
}
Results in:


It becomes the default color for all the values, but you can also define a color for each value.
{
BasicLabel.name: string
name: "Type",
LabelValueOptions.color?: Color | undefined
color: "green",
LabelSet.values: (string | [string, LabelValueOptions & {
valueColor?: Color;
}])[]
values: [["Bug", {LabelValueOptions.color?: Color | undefined
color: "red"}], "Feature", "Task"],
}
Results in:



The supported values syntax for colors and examples are:
- Atlassian theme colors:
purple
,purpleLight
… - Atlassian palette colors:
Blue100
,Blue200
,Red100
… - HEX:
#ff0000
- RGB:
rgb(255, 0, 0)
- RGBA:
rgba(255, 0, 0, 0.5)
- HSL:
hsl(0, 100%, 50%)
- HSLA:
hsla(0, 100%, 50%, 0.5)
Icons and Unicode
Flowie labels have support for Unicode. You define your label as:
{
BasicLabel.name: string
name: "Performance 🚀",
LabelValueOptions.color?: Color | undefined
color: "Magenta200",
}
Results in:

Removing or renaming labels from the schema
Once a label is removed from the schema it will not be shown in the pull request. However, the label will still be present in the pull request and if you add it back to the schema, it will be shown again.
Currently, there is no way to rename a label. If you want to rename a label, you must define a new one, add it to the pull request and remove the previous value. If you just change the label name, Flowie will treat it as a new label.
A complete example
import {const configure: (config: Config | (() => Config)) => void
configure} from "flowie.app"
function configure(config: Config | (() => Config)): void
configure({
Config.labels?: {
schema?: Array<Label>;
} | undefined
labels: {
schema?: Label[] | undefined
schema: [
{BasicLabel.name: string
name: "My label"},
{BasicLabel.name: string
name: "Priority", LabelValueOptions.color?: Color | undefined
color: "Purple100", LabelSet.values: (string | [string, LabelValueOptions & {
valueColor?: Color;
}])[]
values: ["Low", "Medium", "High"]},
{
BasicLabel.name: string
name: "Type",
LabelSet.values: (string | [string, LabelValueOptions & {
valueColor?: Color;
}])[]
values: [
["Bug", {LabelValueOptions.color?: Color | undefined
color: "red"}],
["Feature", {LabelValueOptions.color?: Color | undefined
color: "teal"}],
],
},
],
},
})