Branch sync plugin
This plugin has the same functionality of the basic check maximumCommitsBehind, but adds more options and features:
- Sync using rebase or merge
- Automatic sync
- A merge check that enforces linear history via rebase
- Support for preventing redundant builds
To enable it, use the following config:
ts
import {configure } from "flowie.app"import {branchSync } from "flowie.app/plugins"configure ({plugins : [branchSync ({using : "merge"})],})
Options:
using
- The strategy to be used: rebase
or merge
. Required.
maximumCommitsBehind
- Maximum number of commits behind the destination branch to enforce.
Default: 0
auto
- Automatically syncs the branch
when it gets behind by maximumCommitsBehind
commits.
Default: false
allow
- The groups that are allowed to perform the fix actions. Supports @author
. Default: Anyone that has write permissions is allowed.
enforceLinearHistory
- Requires that the branch has no merge commits and is not behind the destination branch. It also adds an action to rebase and fix the check. Default: false
preventRedundantBuilds
- Applies redundant builds prevention when performing the sync. Default: true
Enforcing linear history
Typically, when sharing a feature branch with other members of the team,
you will want to sync using merge
and then rebase
once it’s ready to merge into the destination.

Because b9e3da3
is a merge commit,
Flowie creates a failing merge check with a ‘Rebase’ action
to allow this to be fixed.
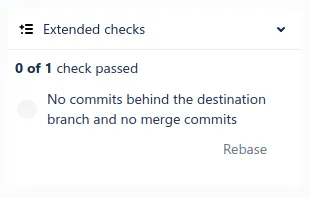
The enforceLinearHistory
option can enforce this workflow:
ts
import {configure } from "flowie.app"import {branchSync } from "flowie.app/plugins"configure ({plugins : [branchSync ({using : "merge",enforceLinearHistory : true,}),],})
Keep in sync automatically
Flowie can keep your feature branch in sync with the destination automatically, so you and your team members can just pull from remote.
ts
import {configure } from "flowie.app"import {branchSync } from "flowie.app/plugins"configure ({plugins : [branchSync ({using : "merge",auto : true,}),],})
Additionally, keep the branch in sync by rebasing it while it’s waiting to be merged. So it is always ready to be merged:
ts
import {configure } from "flowie.app"import {branchSync } from "flowie.app/plugins"import {labels ,otherwise } from "flowie.app/conditions"configure ({labels : {schema : [{name : "Auto sync"}],},plugins : [branchSync ({auto :labels .has ("Auto sync"),using : [[labels .has ("Draft"), "merge"],[otherwise , "rebase"],],enforceLinearHistory : true,}),],})
With this config, Flowie will always keep the branch in sync by merging the destination into the feature branch, and once it is no longer a Draft pull request, it will start rebasing it.